Add Login System in Iframe Implementation
Here’s a diagram showing the basic structure of the login system implementation:
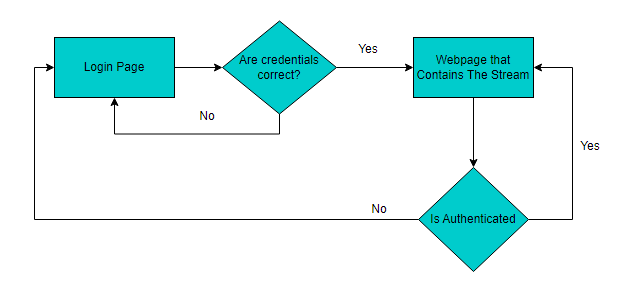
First, you need a login page with a form:
CODE<form onsubmit="handleLogin(event)"> <h3>Login Here</h3> <label for="username">Username</label> <input type="text" placeholder="Email or Phone" id="username" name="username"> <label for="password">Password</label> <input type="password" placeholder="Password" id="password" name="password"> <button type="submit">Log In</button> </form>
On form submission, you need to do your authentication logic:
CODEfunction handleLogin(e){ e.preventDefault(); const form = e.target; const username = form.username.value; const password = form.password.value; //your login logic goes here localStorage.setItem("isAuthenticated", true); window.location.href = "/E3DS_Iframe_Demo.htm"; //Redirect to the main webpage }
You should also check if the user is logged in on the webpage containing the stream. If the user is not logged in, redirect to the login page:
CODEwindow.onload = function(){ if(!localStorage.getItem("isAuthenticated")){ //replace this with your authentication check logic window.location.href = "/login.html" } }