Embedding Stream into Webpage using a no iframe solution
Learn to develop a customized version of the frontend and host it using your own hosting method.
Instructions
We will integrate ‘E3DS core library’ into a demo frontend to take care of all the complex streaming side operations.
To make the system work, you should have an Eagle 3D User Account, Upload an App, and create a Config in the Control Panel.
demo Frontend Repo : https://github.com/e3ds/full-html-control/tree/html/ejs
This whole minimal version of source code can be found in the branch:
https://github.com/e3ds/streaming_ui/tree/demo-static_token
File name is : minimal.html
It might take some time to load if it is the first load after a recent app update have been uploaded. Also there could be many other factors that's why we need to show a progress bar and other UI things to keep users entertained during the process of loading the stream.
Refer to this document to customize the Loading screen with 2D Assets (for a better Loading experience) and to this document to customize it with a 3D Asset (i.e. video Asset).
We have provided a full example with all required UI elements in the same branch with the file name:
full.html
In the future, we will add a more detailed explanation of all these UI elements.
Method 2:
Manually generating tokens for each visitor becomes impractical for production.
So you have to generate the Token automatically upon each visit.
So we have provided a node js App that uses our token API, which can be found in this branch:
https://github.com/e3ds/full-html-control/tree/html/ejs
1. Use the following command to directly clone the branch:
git clone -b html/ejs --single-branch https://github.com/e3ds/full-html-control
2. Open tokenServer.js
3. You have to replace the following info inside tokenServer.js
:
const apiKey = "your streaming api key" // collect from https://account.eagle3dstreaming.com/streaming-api-keys-management
const tokenExpiryDuration = 60000
var clientUserName = "your user name";
var streamingAppInfo =
{
"core": {
"domain": "your domain",
"userName": clientUserName,
"appName": "your app name",
"configurationName": "your config name"
}
}
4. You can collect streaming API Key from this URL:
https://account.eagle3dstreaming.com/streaming-api-keys-management
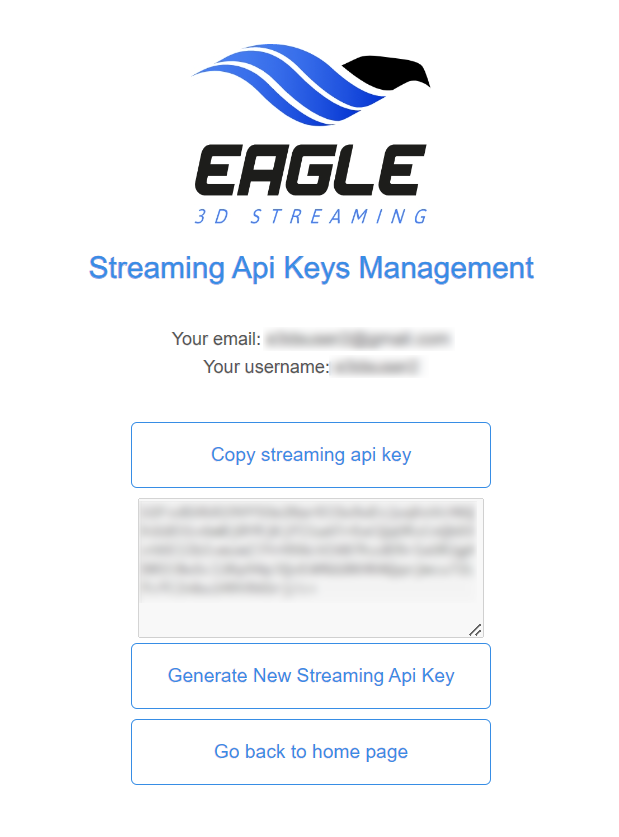
5. If you are using production system then domain
would be “connector.eagle3dstreaming.com“. If you are provided with a dedicated system, then domain
would be the domain of that dedicated system.
If you are on windows then:
6. first, install node js
Run setup.bat
Then run startTokenServer.bat
open browser and in address bar put :
https://localhost:55555/testToken
If all the information is provided correctly, the session will start automatically.
Calling Token Generating API in the front-end exposes your Streaming API Key. Although it can’t be used to delete your apps or replace them with different apps, someone can misuse it to stream your apps without your permission. In such cases Eagle 3D Streaming will not be responsible. To avoid such incident we suggest calling the Token Generating API from the Back-end. And passing that token to the Front-end by server side rendering technique.
Useful Documents for Adding Multiple App and Config Support
See this document for adding multiple app and config support in no-iframe implementation.
Useful Global Functions
See this document for the useful Global Functions.
Need help? Contact Support
Submit a new request at E3DS support portal.
Requests sent on weekends will not be addressed until the following business day.